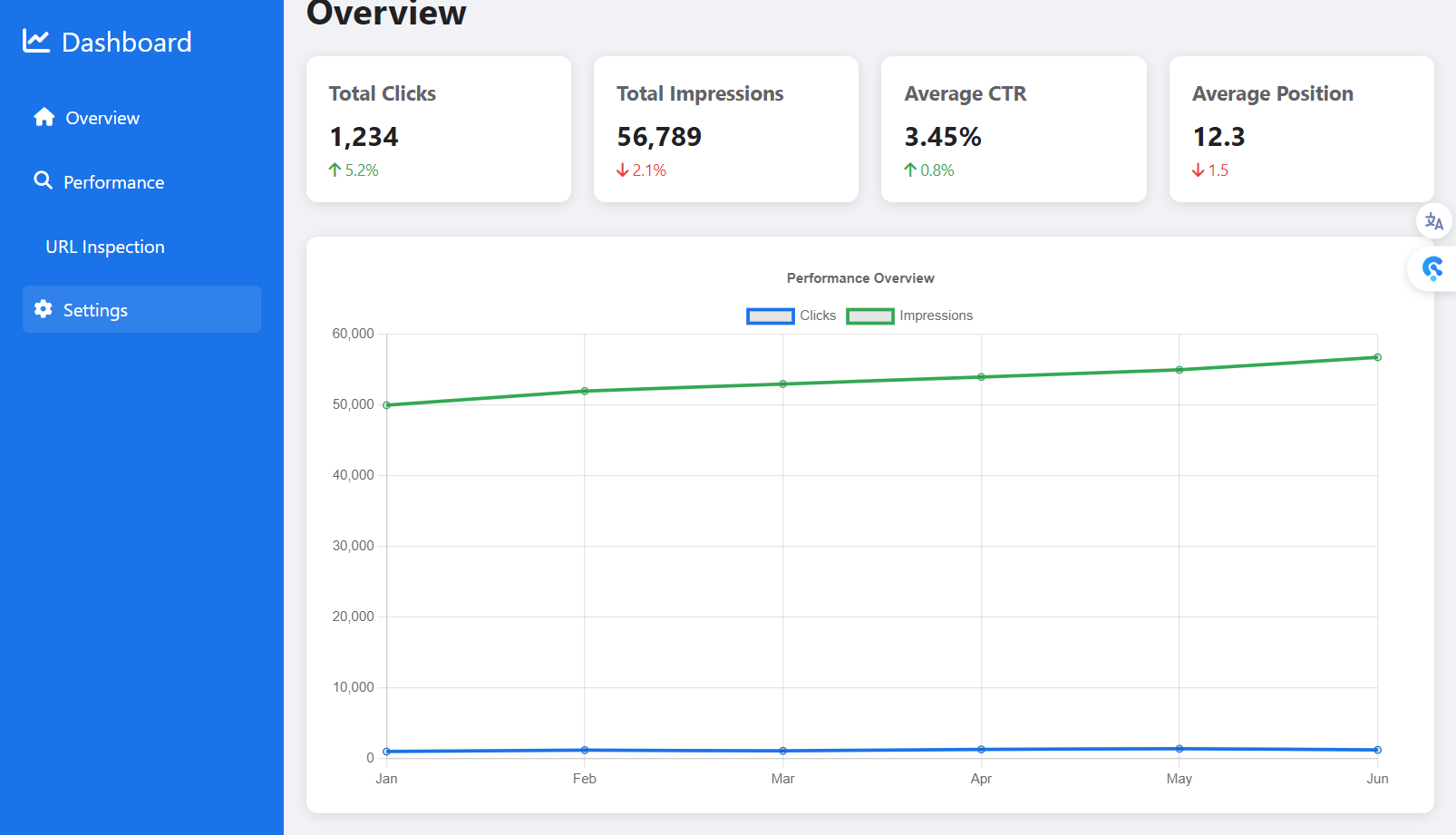
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Modern Dashboard</title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.4.0/css/all.min.css">
<link rel="stylesheet" href="style.css">
</head>
<div class="container">
<nav class="sidebar">
<div class="logo">
<i class="fas fa-chart-line"></i>
<span>Dashboard</span>
</div>
<ul>
<li class="active"><a href="#"><i class="fas fa-home"></i> Overview</a></li>
<li><a href="#"><i class="fas fa-search"></i> Performance</a></li>
<li><a href="#"><i class="fas fa-url"></i> URL Inspection</a></li>
<li><a href="#"><i class="fas fa-cog"></i> Settings</a></li>
</ul>
</nav>
<main>
<header>
<div class="search-bar">
<input type="text" placeholder="Search...">
<i class="fas fa-search"></i>
</div>
<div class="user-info">
<i class="fas fa-bell"></i>
<img src="https://via.placeholder.com/40" alt="User Avatar">
</div>
</header>
<div class="dashboard-content">
<h1>Overview</h1>
<div class="stats-container">
<div class="stat-card">
<h3>Total Clicks</h3>
<p class="stat-value">1,234</p>
<p class="stat-change positive"><i class="fas fa-arrow-up"></i> 5.2%</p>
</div>
<div class="stat-card">
<h3>Total Impressions</h3>
<p class="stat-value">56,789</p>
<p class="stat-change negative"><i class="fas fa-arrow-down"></i> 2.1%</p>
</div>
<div class="stat-card">
<h3>Average CTR</h3>
<p class="stat-value">3.45%</p>
<p class="stat-change positive"><i class="fas fa-arrow-up"></i> 0.8%</p>
</div>
<div class="stat-card">
<h3>Average Position</h3>
<p class="stat-value">12.3</p>
<p class="stat-change negative"><i class="fas fa-arrow-down"></i> 1.5</p>
</div>
</div>
<div class="chart-container">
<canvas id="performanceChart"></canvas>
</div>
</div>
</main>
</div>
<script src="https://cdn.jsdelivr.net/npm/chart.js"></script>
<script src="/app.js"></script>
</body>
</html>
// Variables
$text-color: #202124; // Define the text color
$sidebar-bg: #1a73e8; // Sidebar background color
$body-bg: #f0f2f5; // Body background color
$input-bg: #e8eaed; // Input background color
$card-bg: white; // Card background color
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
font-family: "Segoe UI", Tahoma, Geneva, Verdana, sans-serif;
background-color: $body-bg;
}
.container {
display: flex;
height: 100vh;
.sidebar {
width: 250px;
background-color: $sidebar-bg;
color: white;
padding: 20px;
.logo {
display: flex;
align-items: center;
font-size: 24px;
margin-bottom: 30px;
i {
margin-right: 10px;
}
}
ul {
list-style-type: none;
li {
margin-bottom: 15px;
a {
color: white;
text-decoration: none;
display: flex;
align-items: center;
padding: 10px;
border-radius: 5px;
transition: background-color 0.3s;
i {
margin-right: 10px;
}
}
&.active a,
&:hover a {
background-color: rgba(255, 255, 255, 0.1);
}
}
}
}
main {
flex-grow: 1;
padding: 20px;
overflow-y: auto;
header {
display: flex;
justify-content: space-between;
align-items: center;
margin-bottom: 30px;
.search-bar {
position: relative;
width: 300px;
input {
width: 100%;
padding: 10px 15px;
border: none;
border-radius: 20px;
background-color: $input-bg;
}
i {
position: absolute;
right: 15px;
top: 50%;
transform: translateY(-50%);
color: #5f6368;
}
}
.user-info {
display: flex;
align-items: center;
i {
margin-right: 20px;
font-size: 20px;
color: #5f6368;
}
img {
width: 40px;
height: 40px;
border-radius: 50%;
}
}
}
.dashboard-content {
h1 {
margin-bottom: 20px;
color: $text-color;
}
.stats-container {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(200px, 1fr));
gap: 20px;
margin-bottom: 30px;
.stat-card {
background-color: $card-bg;
border-radius: 10px;
padding: 20px;
box-shadow: 0 2px 10px rgba(0, 0, 0, 0.1);
h3 {
font-size: 18px;
color: #5f6368;
margin-bottom: 10px;
}
.stat-value {
font-size: 24px;
font-weight: bold;
color: $text-color;
margin-bottom: 5px;
}
.stat-change {
font-size: 14px;
&.positive {
color: #34a853;
}
&.negative {
color: #ea4335;
}
}
}
}
.chart-container {
background-color: $card-bg;
border-radius: 10px;
padding: 20px;
box-shadow: 0 2px 10px rgba(0, 0, 0, 0.1);
}
}
}
}
// Performance
const ctx = document.getElementById('performanceChart').getContext('2d');
new Chart(ctx, {
type: 'line',
data: {
labels: ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun'],
datasets: [{
label: 'Clicks',
data: [1000, 1200, 1100, 1300, 1400, 1234],
borderColor: '#1a73e8',
tension: 0.1
}, {
label: 'Impressions',
data: [50000, 52000, 53000, 54000, 55000, 56789],
borderColor: '#34a853',
tension: 0.1
}]
},
options: {
responsive: true,
plugins: {
legend: {
position: 'top',
},
title: {
display: true,
text: 'Performance Overview'
}
},
scales: {
y: {
beginAtZero: true
}
}
}
});
// Add interactivity to sidebar menu items
const menuItems = document.querySelectorAll('.sidebar ul li');
menuItems.forEach(item => {
item.addEventListener('click', () => {
menuItems.forEach(i => i.classList.remove('active'));
item.classList.add('active');
});
});
Modern Dashboard Description
The Modern Dashboard is a sleek and responsive web interface designed to provide users with a comprehensive overview of key performance metrics. Its layout is organized into a sidebar navigation and a main content area, ensuring ease of use and accessibility.
Structure Overview
- HTML Structure:
- The document begins with standard meta tags for character set and viewport settings, ensuring compatibility across devices.
- A title tag identifies the page as “Modern Dashboard.”
- External Resources:
- The design utilizes Font Awesome for scalable vector icons, enhancing visual communication within the interface.
- A stylesheet (
style.css
) is linked to apply custom styles.
- Container:
- The main container uses a flexbox layout to distribute space between the sidebar and the main content.
- Sidebar Navigation:
- The sidebar features a branding section with a logo icon and title.
- It contains a vertical navigation menu with links for different sections: Overview, Performance, URL Inspection, and Settings.
- The active link is highlighted for user guidance.
- Main Content Area:
- The header section includes a search bar for easy access to information and a user info section displaying a notification icon and user avatar.
- Below the header, the main content is divided into:
- Overview Title: Clearly indicates the current section.
- Stats Container: A grid layout displays various performance metrics, each within a stat card. Key metrics include:
- Total Clicks
- Total Impressions
- Average Click-Through Rate (CTR)
- Average Position
- Each metric card shows the title, value, and percentage change, using color coding (green for positive changes and red for negative).
- Chart Container:
- A dedicated area for visual data representation using a
canvas
element, ready for integration with Chart.js for interactive and dynamic charts.
- A dedicated area for visual data representation using a
- Scripts:
- The dashboard includes external JavaScript libraries like Chart.js for data visualization and a custom script (
app.js
) for any additional functionality.
- The dashboard includes external JavaScript libraries like Chart.js for data visualization and a custom script (
primer-8