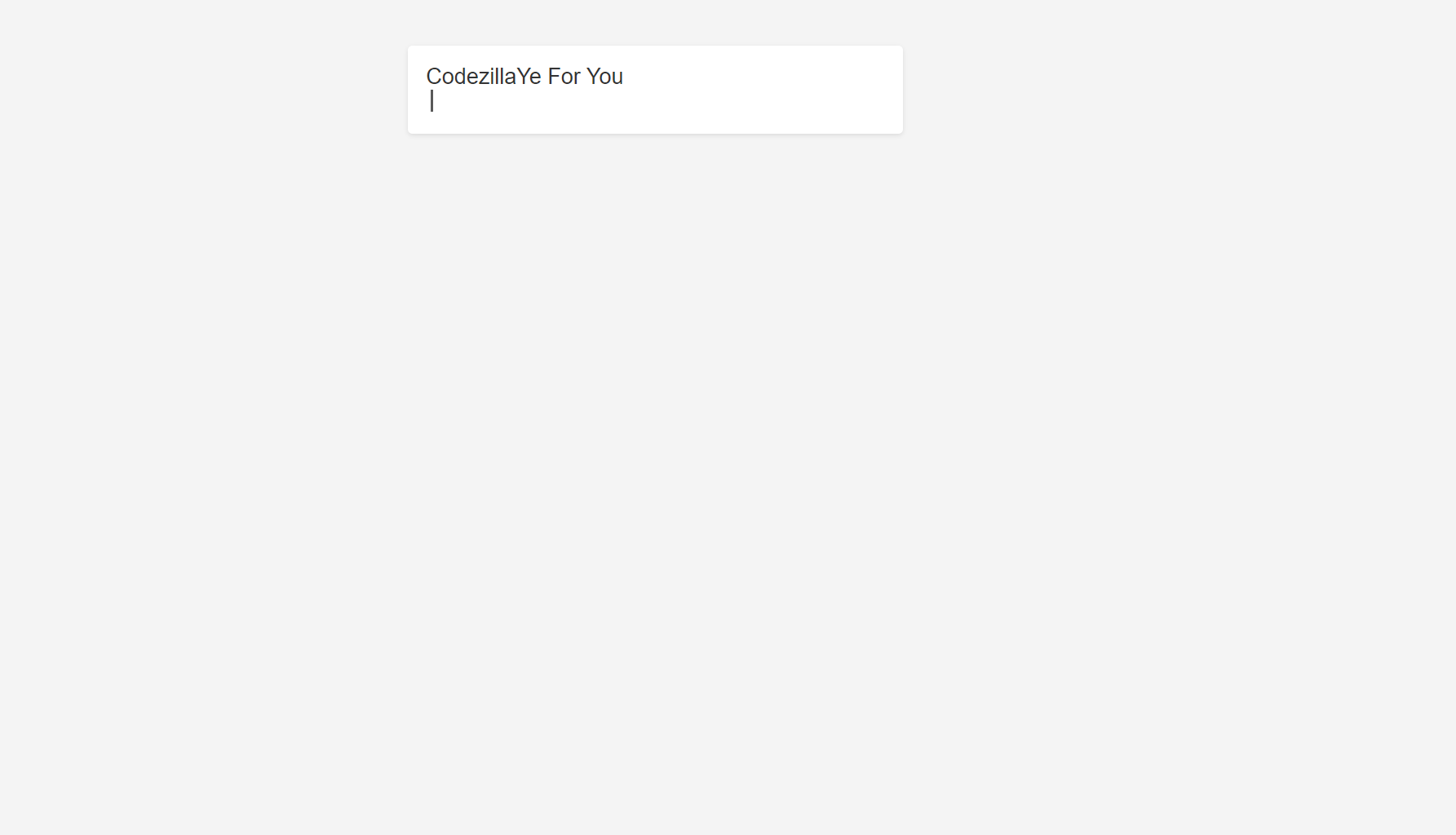
You can View Live code Go to >> [ Live Code Preview/Preview JS ]
<!DOCTYPE html>
<html>
<head>
<title>Auto Text</title>
<style>
body {
margin: 0;
padding: 0;
font-family: sans-serif;
background-color: #f4f4f4;
}
.container {
width: 500px;
margin: 50px auto;
padding: 20px;
background-color: #fff;
border-radius: 5px;
box-shadow: 0 2px 5px rgba(0, 0, 0, 0.1);
}
.text-container {
font-size: 24px;
color: #333;
}
.cursor {
display: inline-block;
width: 3px;
height: 24px;
background-color: #333;
margin-left: 5px;
animation: blink 1s infinite;
}
@keyframes blink {
0% { opacity: 1; }
50% { opacity: 0; }
100% { opacity: 1; }
}
</style>
</head>
<body>
<div class="container">
<div class="text-container" id="auto-text"></div>
<div class="cursor"></div>
</div>
<script>
const text = "CodezillaYe For You";
const autoText = document.getElementById("auto-text");
let index = 0;
let typing = true;
let typingSpeed = 100; // Adjust the speed in milliseconds
function type() {
if (typing) {
autoText.innerHTML += text.charAt(index);
index++;
if (index === text.length) {
typing = false;
setTimeout(erase, 1000); // Pause before erasing
} else {
setTimeout(type, typingSpeed);
}
}
}
function erase() {
if (!typing) {
autoText.innerHTML = text.substring(0, index - 1);
index--;
if (index === 0) {
typing = true;
setTimeout(type, 1000); // Pause before typing again
} else {
setTimeout(erase, typingSpeed);
}
}
}
type();
</script>
</body>
</html>