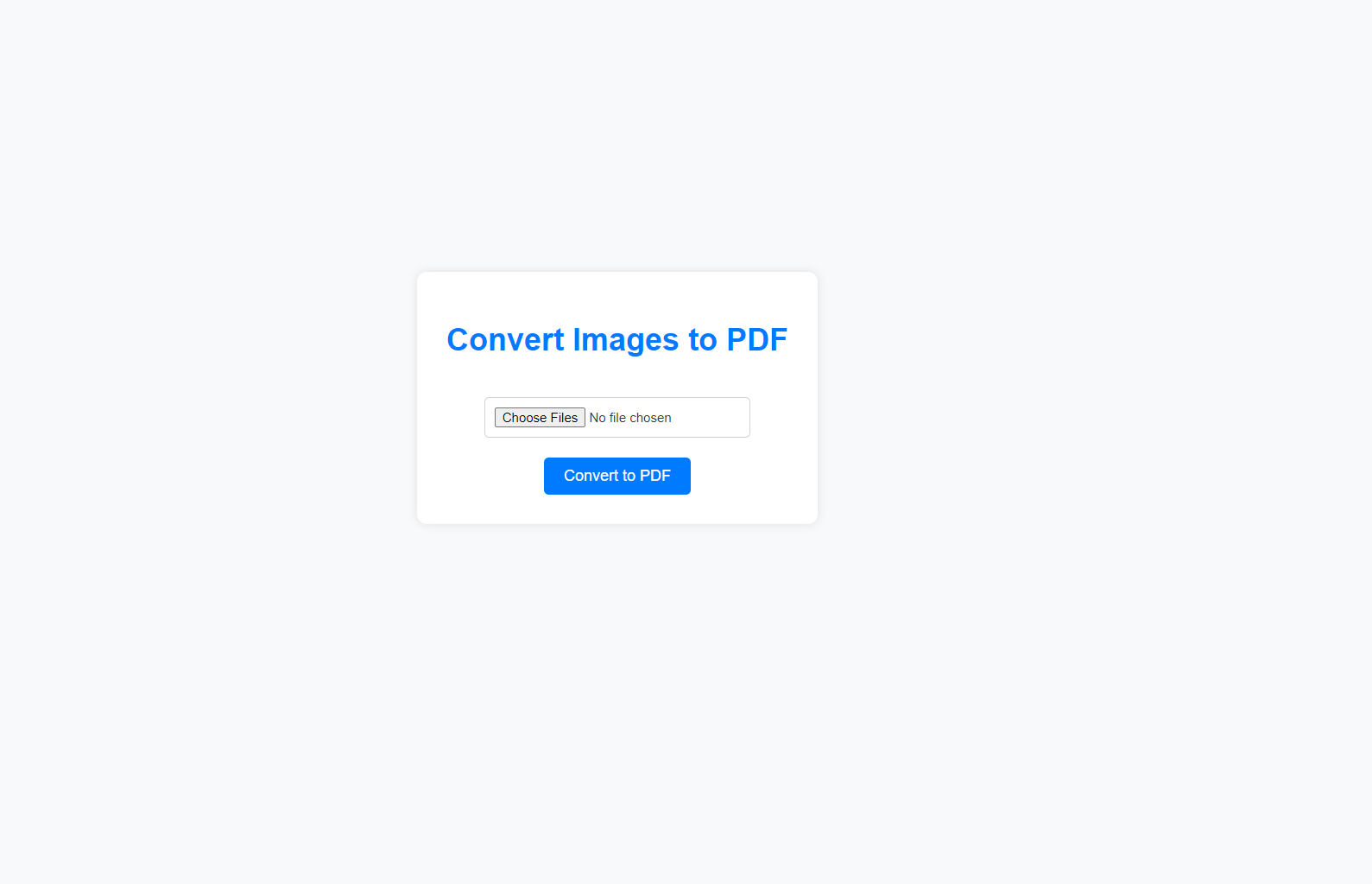
To convert images to a PDF and then collect all images into one file using JavaScript, you can use the jsPDF
library. This library allows you to create PDFs directly from the browser. Here is a step-by-step guide:
Steps to Convert Images to a PDF and Combine Them Using jsPDF
1-Include the jsPDF Library:
**You can include jsPDF
in your HTML file via a CDN.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Convert Images to PDF</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jspdf/2.5.1/jspdf.umd.min.js"></script>
<style>
body {
font-family: Arial, sans-serif;
background-color: #f8f9fa;
color: #343a40;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
height: 100vh;
margin: 0;
}
h1 {
font-size: 2em;
margin-bottom: 20px;
color: #007bff;
}
.container {
background: white;
padding: 30px;
border-radius: 10px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
text-align: center;
}
input[type="file"] {
margin: 20px 0;
padding: 10px;
border: 1px solid #ced4da;
border-radius: 5px;
}
button {
padding: 10px 20px;
background-color: #007bff;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
font-size: 1em;
}
button:hover {
background-color: #0056b3;
}
</style>
</head>
<body>
<div class="container">
<h1>Convert Images to PDF</h1>
<input type="file" id="imageFiles" multiple accept="image/*">
<br>
<button onclick="convertImagesToPdf()">Convert to PDF</button>
</div>
<script>
async function convertImagesToPdf() {
const { jsPDF } = window.jspdf;
const pdf = new jsPDF();
const files = document.getElementById('imageFiles').files;
if (files.length === 0) {
alert("Please select image files.");
return;
}
for (let i = 0; i < files.length; i++) {
const file = files[i];
const imgData = await readFileAsDataURL(file);
if (i > 0) {
pdf.addPage();
}
const img = new Image();
img.src = imgData;
await new Promise((resolve) => {
img.onload = () => {
const width = pdf.internal.pageSize.getWidth();
const height = (img.height * width) / img.width;
pdf.addImage(img, 'JPEG', 0, 0, width, height);
resolve();
};
});
}
pdf.save("combined_images.pdf");
}
function readFileAsDataURL(file) {
return new Promise((resolve, reject) => {
const reader = new FileReader();
reader.onload = () => resolve(reader.result);
reader.onerror = reject;
reader.readAsDataURL(file);
});
}
</script>
</body>
</html>
Explanation:
JavaScript:
- The
convertImagesToPdf
function is called when the button is clicked. - It uses the
jsPDF
library to create a new PDF document. - It reads the selected image files as Data URLs.
- It iterates through the files, adds each image to the PDF, and resizes it to fit the page.
- It saves the final PDF with the combined images.
How to Use:
- Open the HTML file in a browser.
- Select multiple image files using the file input.
- Click the “Convert to PDF” button.
- The combined PDF will be downloaded.
This script uses the
FileReader
API to read image files and thejsPDF
library to create and manipulate the PDF. It ensures each image fits the page width while maintaining the aspect ratio