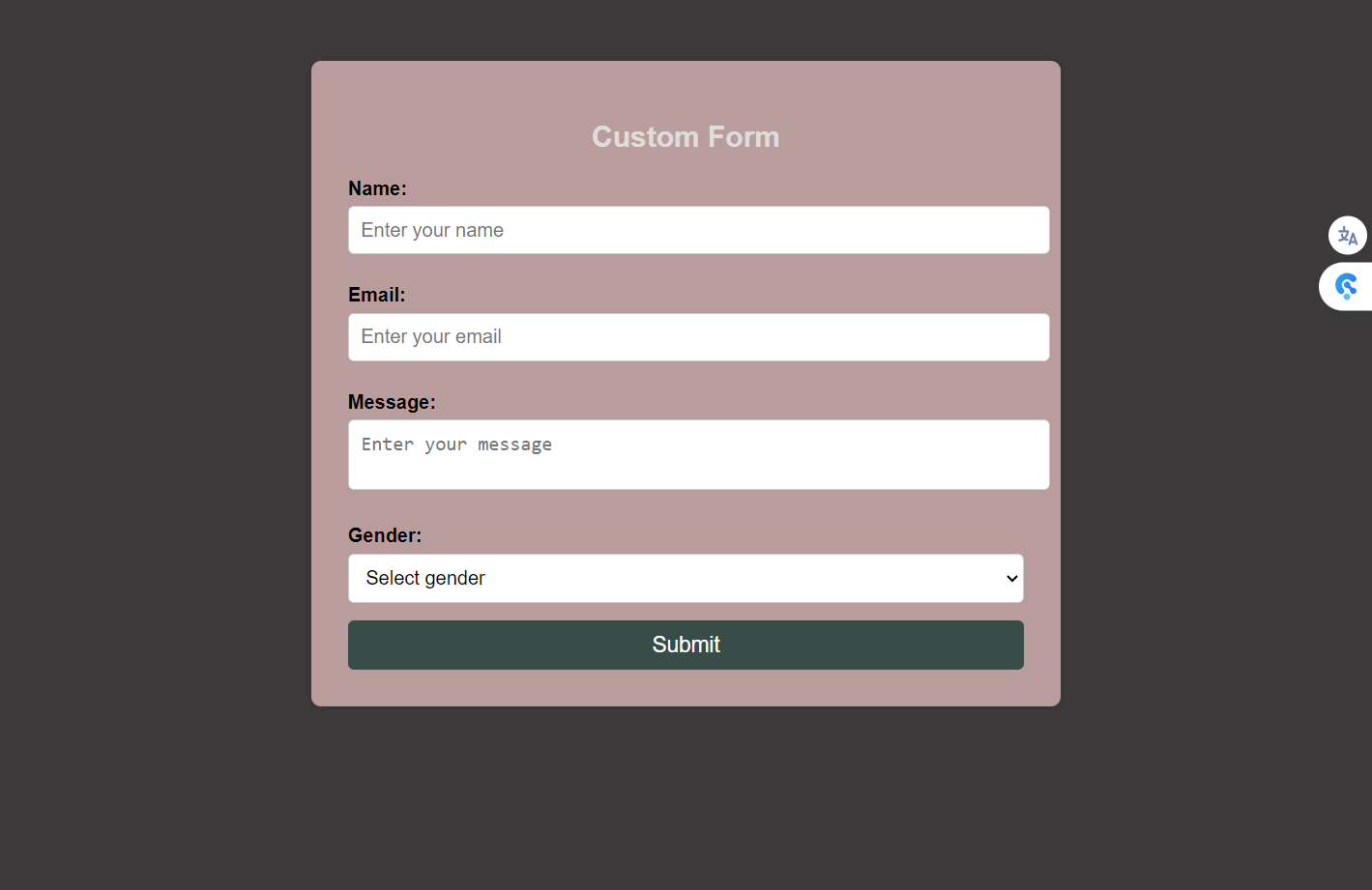
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Custom Form</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="form-container">
<form id="customForm" action="submit.php" method="POST">
<h2>Custom Form</h2>
<label for="name">Name:</label>
<input type="text" id="name" name="name" placeholder="Enter your name" required>
<label for="email">Email:</label>
<input type="email" id="email" name="email" placeholder="Enter your email" required>
<label for="message">Message:</label>
<textarea id="message" name="message" placeholder="Enter your message" required></textarea>
<label for="gender">Gender:</label>
<select id="gender" name="gender" required>
<option value="" disabled selected>Select gender</option>
<option value="male">Male</option>
<option value="female">Female</option>
<option value="other">Other</option>
</select>
<button type="submit">Submit</button>
</form>
</div>
<script src="/app.js"></script>
</body>
</html>
body {
font-family: Arial, sans-serif;
background-color: #3c3a3a;
}
.form-container {
width: 50%;
margin: 50px auto;
background-color: #b99d9d;
padding: 30px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
border-radius: 8px;
}
h2 {
text-align: center;
color: #dfdcdc;
}
label {
font-weight: bold;
display: block;
margin: 10px 0 5px;
}
input[type="text"], input[type="email"], textarea, select {
width: 100%;
padding: 10px;
margin-bottom: 15px;
border: 1px solid #ccc;
border-radius: 5px;
font-size: 16px;
}
button {
width: 100%;
padding: 10px;
background-color: #384d48;
color: #fff;
border: none;
border-radius: 5px;
font-size: 18px;
cursor: pointer;
}
button:hover {
background-color: #8B8149;
}
textarea {
resize: none;
}
document.getElementById('customForm').addEventListener('submit', function(event) {
const name = document.getElementById('name').value;
const email = document.getElementById('email').value;
const message = document.getElementById('message').value;
if (name === "" || email === "" || message === "") {
alert("Please fill out all fields.");
event.preventDefault();
} else {
alert("Form submitted successfully!");
}
});
About Design
Here’s a quick description of the custom form design:
Custom Form Design Overview:
- Structure: The form includes fields for name, email, message, and gender selection, with labels for clarity.
- Layout: The form is placed within a centered container with padding and rounded corners, giving it a clean, modern look.
- Styling: A simple color scheme using #384d48 and #8B8149 for buttons and hover effects. Inputs and buttons are styled with consistent padding, borders, and rounded corners.
- Responsiveness: The form is flexible and adjusts to various screen sizes, using
width: 50%
for desktop andwidth: 100%
on mobile. - Functionality: JavaScript handles basic validation, ensuring all fields are filled before submission.