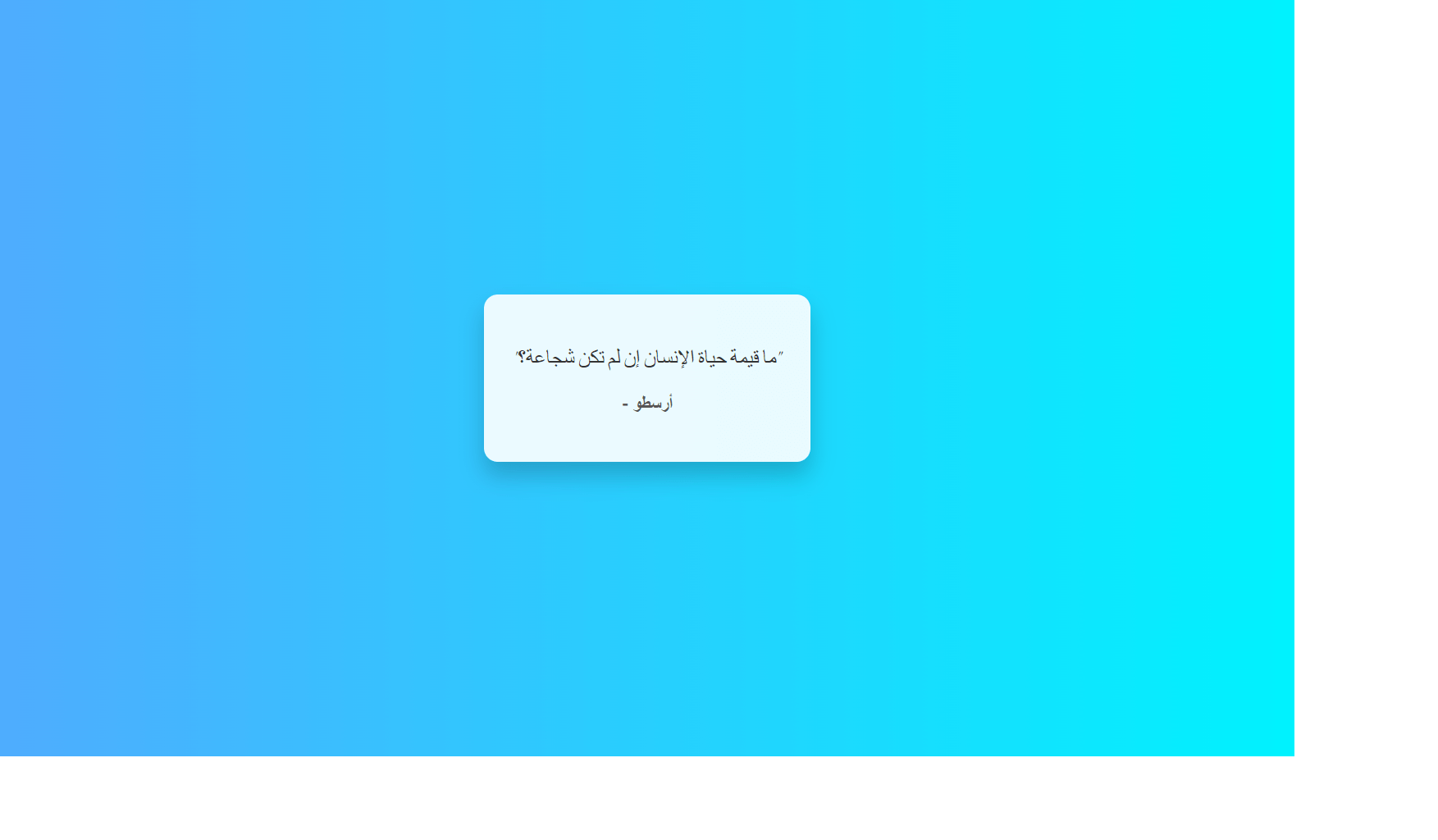
You can also watch the Daily Quotes application on the Live Server. Click on this link to go to the Live Code >>> ( Live Code Preview) 🔜
HTML Code </>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Quote of the Day</title>
<link rel="stylesheet" href="style.css">
<link href="https://fonts.googleapis.com/css2?family=Roboto:wght@400;700&family=Great+Vibes&display=swap" rel="stylesheet">
</head>
<body>
<div class="quote-container">
<div class="quote-box">
<p class="quote-text" id="quoteText">"The only limit to our realization of tomorrow is our doubts of today."</p>
<p class="quote-author" id="quoteAuthor">- Franklin D. Roosevelt</p>
</div>
</div>
<script src="app.js"></script>
</body>
</html>
CSS Code </>.
/* styles.css */
body {
font-family: 'Roboto', sans-serif;
margin: 0;
padding: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background: linear-gradient(to right, #4facfe, #00f2fe);
overflow: hidden;
}
.quote-container {
display: flex;
justify-content: center;
align-items: center;
height: 100%;
width: 100%;
}
.quote-box {
background: rgba(255, 255, 255, 0.9);
padding: 2rem;
border-radius: 15px;
box-shadow: 0 15px 25px rgba(0, 0, 0, 0.2);
max-width: 600px;
text-align: center;
animation: fadeIn 2s ease-in-out;
}
.quote-text {
font-size: 1.5rem;
margin-bottom: 1rem;
font-family: 'Great Vibes', cursive;
color: #333;
}
.quote-author {
font-size: 1.2rem;
font-weight: bold;
color: #555;
}
@keyframes fadeIn {
0% { opacity: 0; transform: translateY(-20px); }
100% { opacity: 1; transform: translateY(0); }
}
JS Code </>.
document.addEventListener('DOMContentLoaded', () => {
const quotes = [
{
text: "العشق ماء الØÙŠØ§Ø©ØŒ والعاشق هو Ø§Ù„Ø±ÙˆØ Ù…Ù† النار.",
author: "الرومي"
},
{
text: "The only limit to our realization of tomorrow is our doubts of today.",
author: "Franklin D. Roosevelt"
},
{
text: "The future belongs to those who believe in the beauty of their dreams.",
author: "Eleanor Roosevelt"
},
{
text: "It does not matter how slowly you go as long as you do not stop.",
author: "Confucius"
},
{
text: "Life is 10% what happens to us and 90% how we react to it.",
author: "Charles R. Swindoll"
},
{
text: "أنت مني، وأنا منك.",
author: "ابن عربي"
},
{
text: "Your time is limited, so don't waste it living someone else's life.",
author: "Steve Jobs"
},
{
text: "ما قيمة ØÙŠØ§Ø© الإنسان إن لم تكن شجاعة؟",
author: "أرسطو"
},
{
text: "ضاق صدري، بما Ø±ØØ¨Øª الأرض.",
author: "أبو العلاء المعري"
}
];
const quoteText = document.getElementById('quoteText');
const quoteAuthor = document.getElementById('quoteAuthor');
const today = new Date().getDate();
const quote = quotes[today % quotes.length];
quoteText.textContent = `"${quote.text}"`;
quoteAuthor.textContent = `- ${quote.author}`;
});